Understanding Functions and the Call Stack: A Beginner's Guide
Explore the essentials of functions and the call stack in programming. Learn how functions break down complex problems and how the call stack manages execution flow. This guide sets the stage for advanced topics, including a future deep dive into recursion.
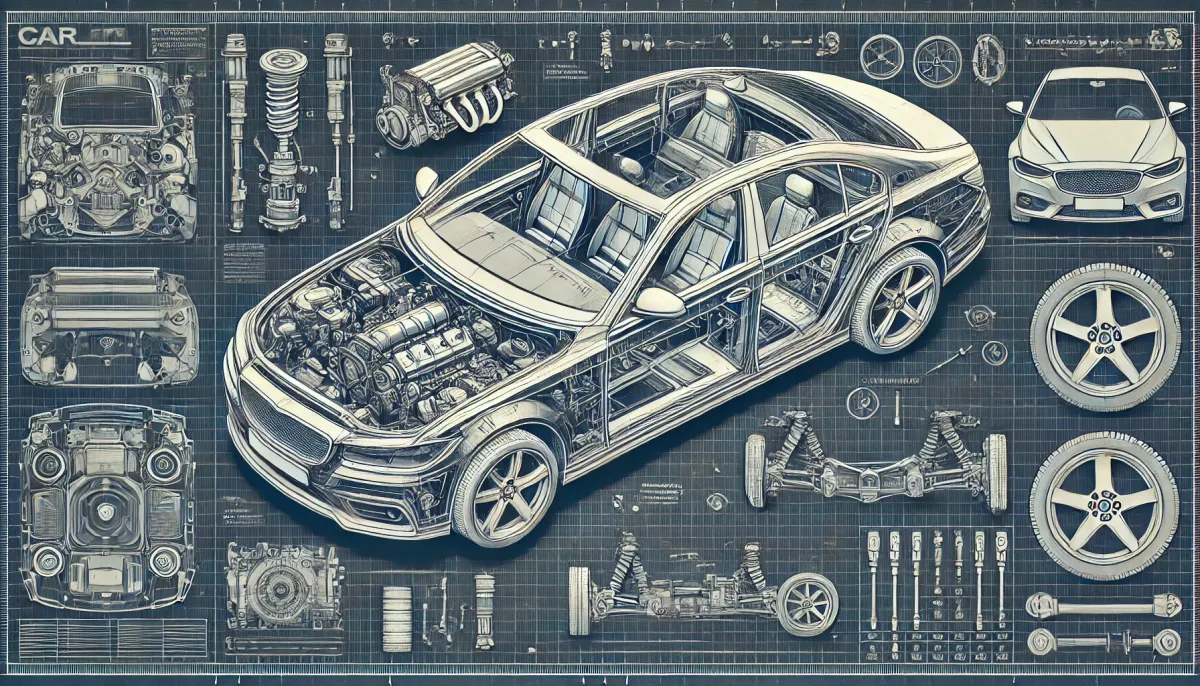
Introduction
In programming, functions and the call stack are foundational concepts that enable developers to write efficient and maintainable code. By breaking complex problems into manageable tasks, functions promote code reusability and improve readability, while the call stack ensures correct execution and flow.
This article was initially planned to cover recursion, a technique that relies heavily on these concepts. However, to provide a more focused exploration, I've decided to dedicate an entire follow-up article to recursion. Here, we'll focus on building a solid understanding of functions and the call stack, setting the stage for diving into recursion later.
In this guide, we’ll explore:
- Functions: The Building Blocks of Programs
We'll start by examining what functions are and how they help structure code effectively. - Function Calls Under the Hood
Next, we'll look at how function calls are managed in a program, introducing the call stack's role. - The Call Stack: An Essential Component
We'll explain how the call stack manages function execution, using analogies to illustrate its operation.
Functions: The Building Blocks of Programs
What are Functions?
Functions are essential in programming, serving as mini-programs within a main program. They allow developers to break down complex tasks into smaller, manageable pieces, enhancing code organization and readability. Let's explore what functions are and why they're so crucial.
Function as a Box
Let's for a moment imagine a function as a box. Inside that box, some magic happens – that's what the function does, its magic.
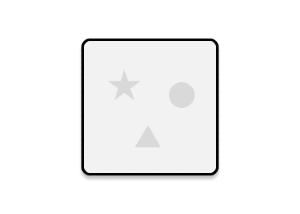
Sometimes, those boxes need something from the outside world to do their magic. Allow me to make another analogy–let's imagine a car.
Think of the car as a complete program. Each component, such as the engine or ignition, is like a function with a distinct responsibility. This modular approach allows each part to function independently while contributing to the overall operation of the car.
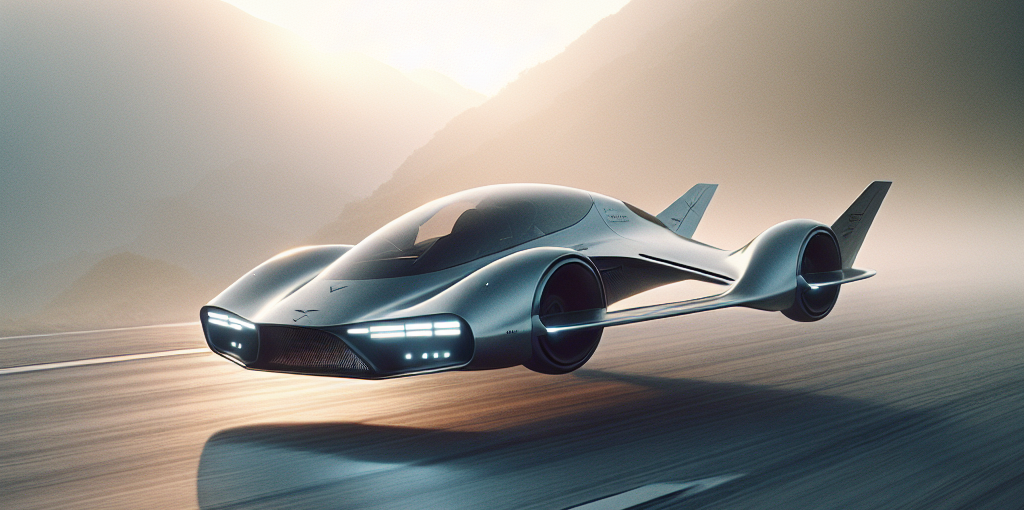
If we think of this car as being our entire program, we can break it down into several smaller components, modules, or functions if you will. Each one of them has a well-defined set of properties and responsibilities:
- Engine
- Suspension
- Ignition
- Braking System
And the list goes on... Each of these "macro" components can be further broken down into even smaller components with more specialized functions.
Parameters
Some functions need information from the "outside world" to perform their tasks. These inputs are called parameters. For example, an ignition system requires a key (input) to start the engine. Similarly, in programming, functions take inputs, perform their tasks, and often return a result.
If we now go back to our function-as-a-box analogy, it would look like this:
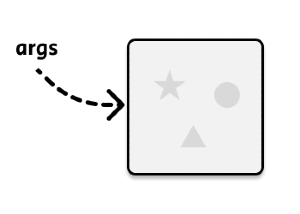
Returning Values
In programming, when a function completes its task, it often returns a value to the part of the program that called it.
The ability to return values makes functions powerful tools for building complex applications, allowing them to communicate results and maintain data flow across the program.
Just as some components of a car need inputs to function, they also might produce outputs after performing their tasks.
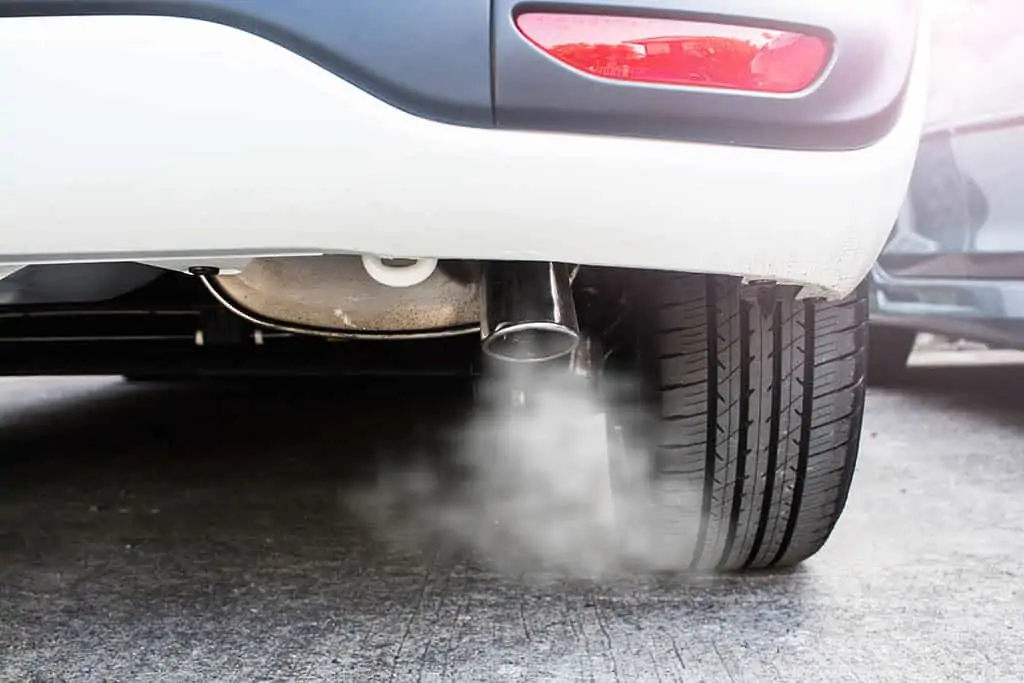
For instance, the engine takes in two parameters–fuel and air–as inputs and produces exhaust gases as the output, just as a function returns a result after computation.
Functions in programming take inputs (parameters), perform their tasks, and then return outputs (results).
Let's look at a simple function that adds two numbers and returns the result:
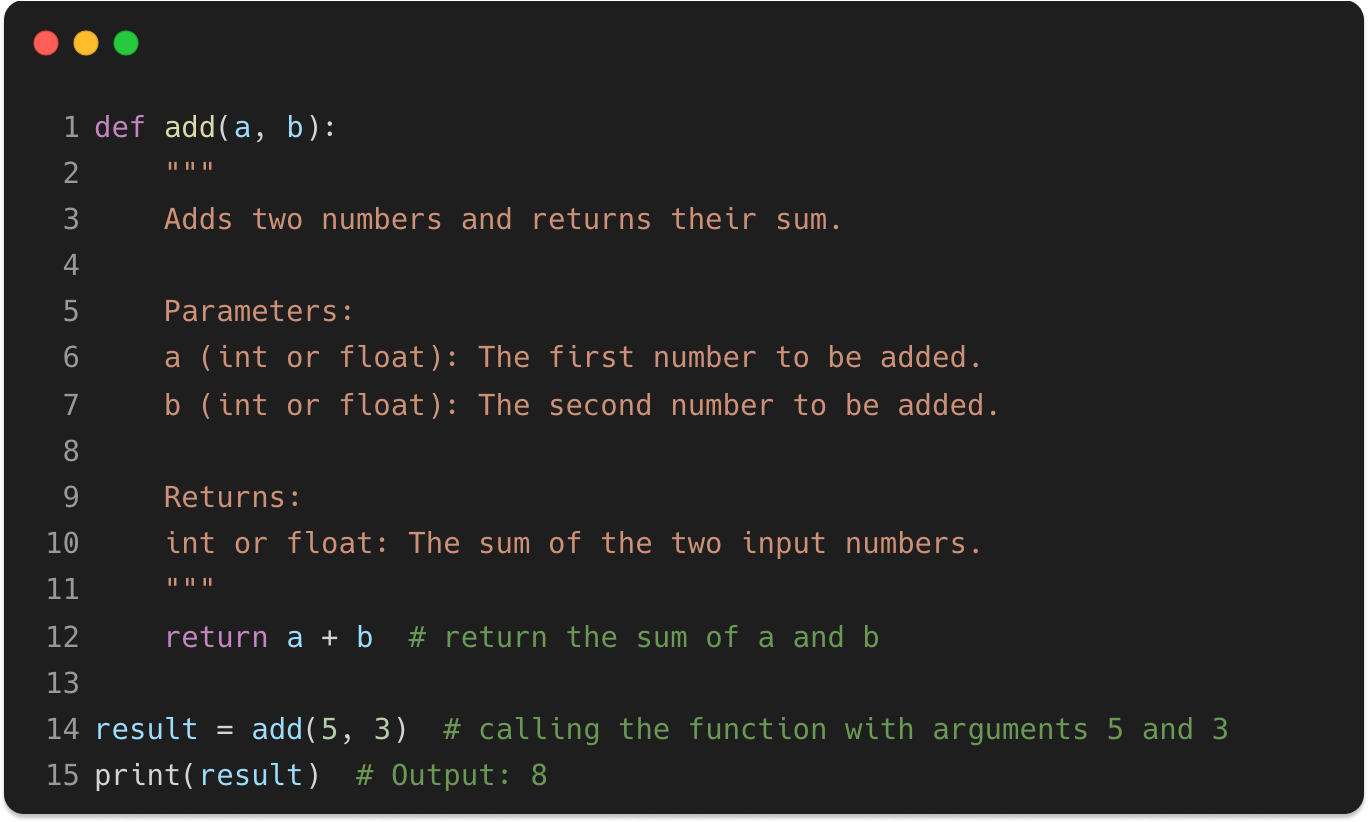
In this example:
- The function
add
takes two inputs (a
andb
). - It performs the task of adding these two numbers.
- It returns the result of the addition to the caller.
To visualize this, let's go back to our function-as-a-box analogy. Here, the box not only takes inputs but also produces an output that is sent back to the outer world.
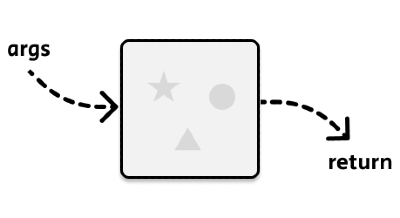
After all that preamble, you might be still asking yourself...
Why Do We Use Functions?
Short answer: Modularity!
Modularity is a core principle in software development that allows you to divide a program into smaller, manageable sections. This makes it easier to understand, maintain, and develop software efficiently.
Using functions allows us to break down a large problem into smaller, manageable, easier to understand pieces.
To sum up, here are some key reasons of why we use functions:
- Reusability: Write once, use multiple times.
- Readability: Makes code easier to read and understand.
- Maintainability: Easier to update and debug.
Functions not only improve the organization of code but also interact with each other in complex ways. This leads us to an important concept: understanding how these interactions are managed in memory, specifically through the call stack.
Function Calls Under The Hood
Now that we've explored why we use functions and how they contribute to modularity in software development, let's dive deeper into how function calls work under the hood. Understanding this process requires a look at the call stack, a fundamental data structure that manages function calls and returns in a program. But before we discuss the call stack, let's take a closer look at the concept of a stack itself.
Stack: The Data Structure
A stack is a fundamental data structure in computer science, characterized by its Last In, First Out (LIFO) behavior. Imagine a stack as a series of plates piled on top of each other. You can only add a new plate to the top, and when you need a plate, you take the top one off. This simple yet powerful structure is widely used in programming for managing tasks that need to be executed in a specific order.
A stack supports two primary operations:
- Push: Adding an element to the top of the stack.
- Pop: Removing the top element from the stack.
This behavior is ideal for situations where the order of operations matters, such as in tracking function calls and handling undo mechanisms in software applications.
Let's illustrate this with an everyday example: your web browser's history. Each time you visit a new webpage, your browser pushes the current page to a stack. If you decide to navigate back, the browser "pops" the current page off the stack and takes you back to the previous one.
Here's a quick demonstration of this concept:
- Visiting a Website: You open a new tab and visit apple.com. At this stage, the stack is empty and the back button is disabled.
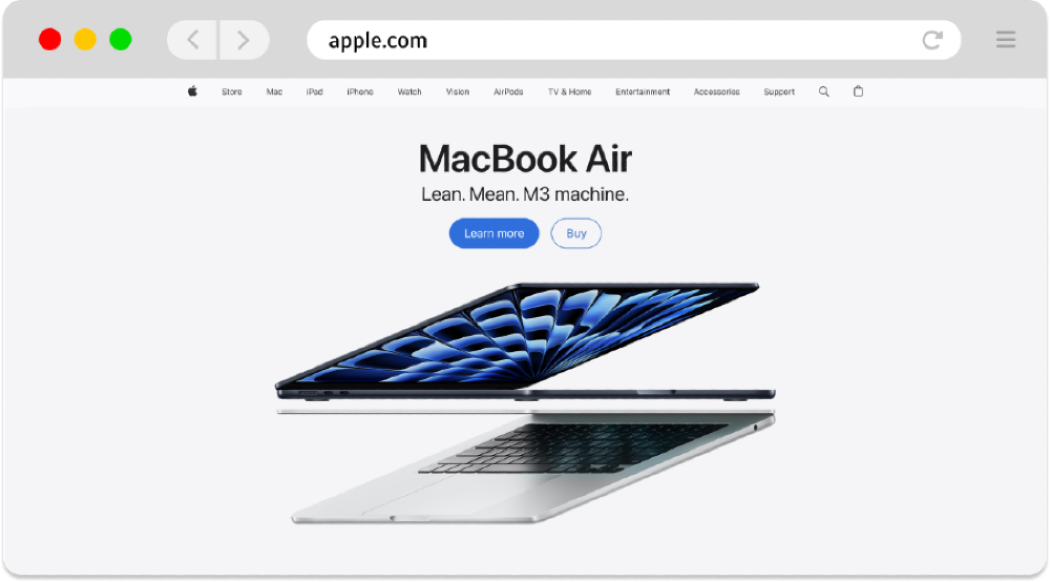
- Navigating to a New Page: You navigate to google.com. Now, apple.com is added to the stack.
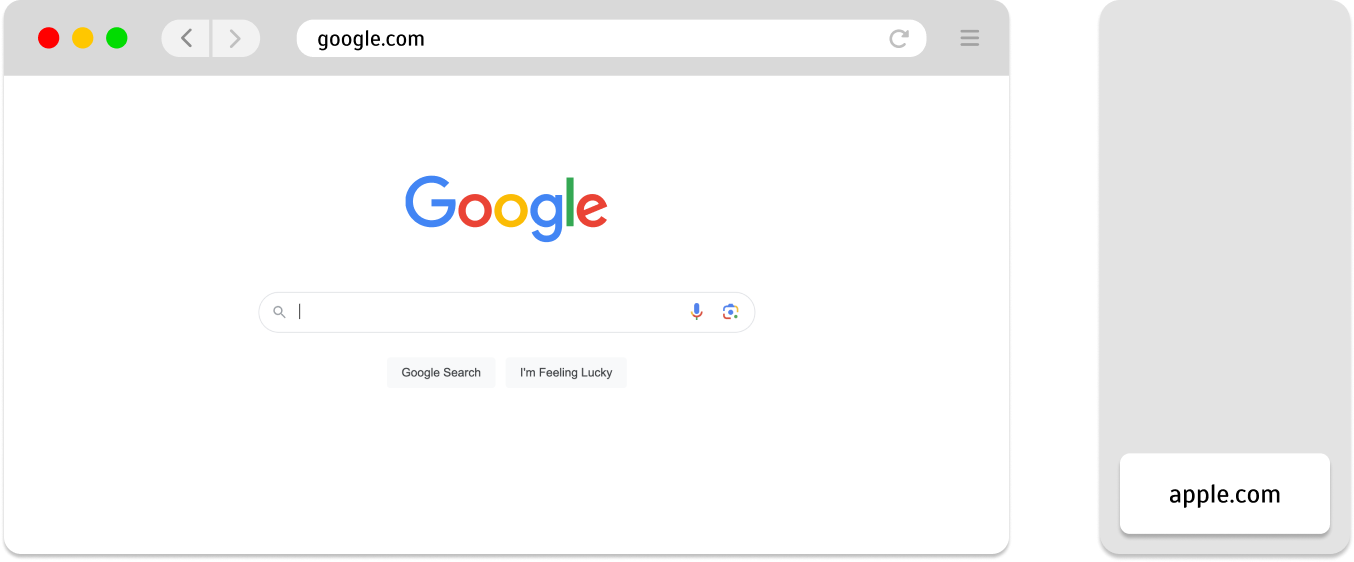
- Executing a Search: You then search for "stack data structure" on Google. This action pushes the Google search page to the top of the stack, maintaining the order of your browsing history.
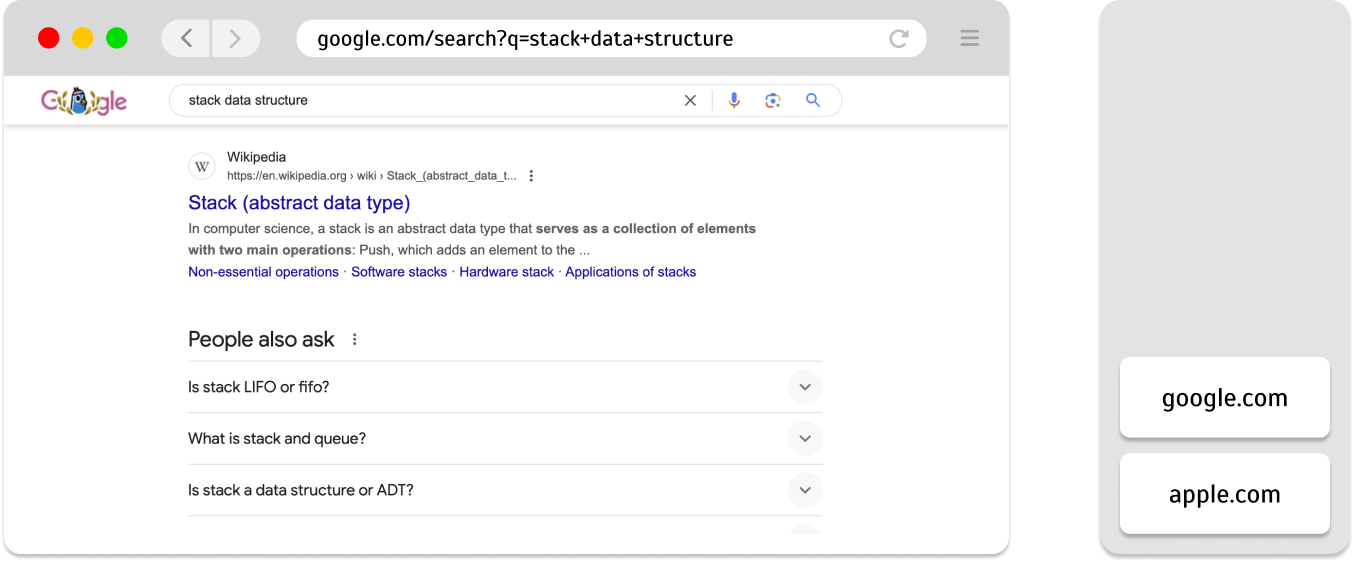
- Navigating Back: If you hit the back button, the stack performs a pop operation. The current page (google.com) is removed, and you're taken back to this page.
This action is illustrated in the image below:
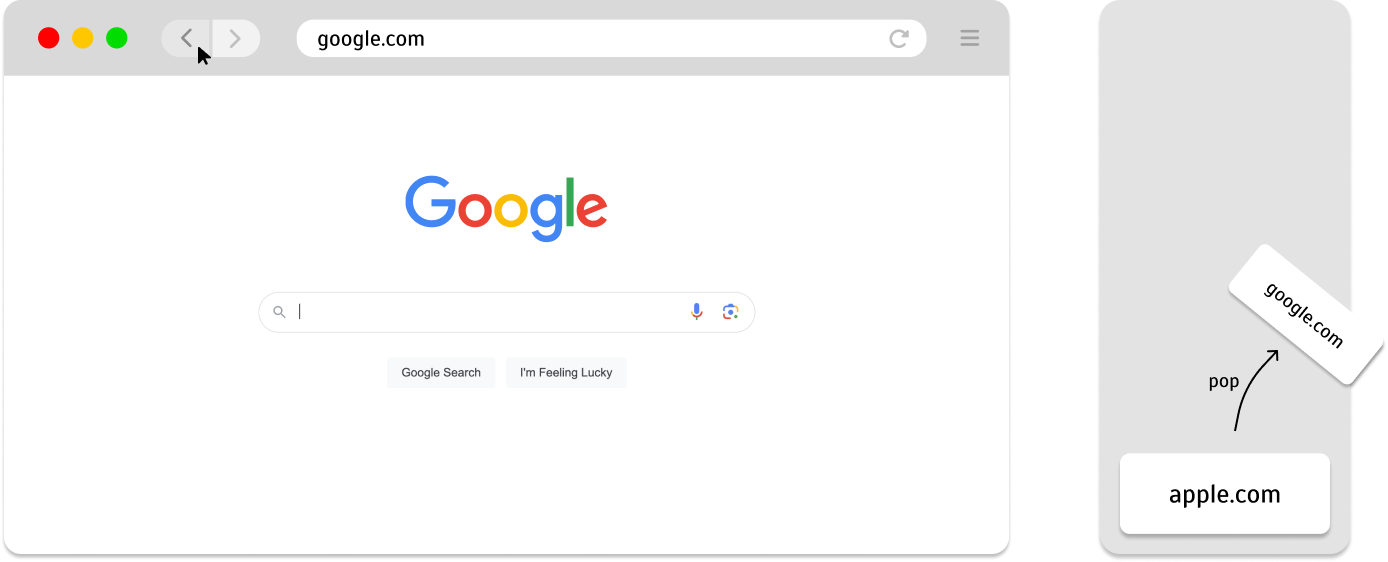
In this image, the left side represents the browser interface as you navigate back, while the right side shows the stack's behavior, where google.com is popped off the stack, revealing apple.com beneath it.
Each browser tab maintains its own stack to keep track of visited pages, ensuring that you can move back and forth seamlessly without losing your place.
The concept of a stack is not limited to web browsing. In the realm of programming, it plays a crucial role in managing function calls. This leads us to the next topic: the call stack.
The Call Stack: An Essential Component
In programming, managing function calls and returns is a crucial task. This is where the call stack comes into play, acting as a mechanism to keep track of multiple function calls and ensuring they execute in the correct order.
Think of the call stack as a save system in a video game. When you reach a checkpoint or pause the game, your current state is saved. This includes your character's position, health, inventory, and any tasks you've completed. Similarly, when a function is called in a program, a stack frame is created to store the current state of that function. This includes:
- Function Arguments: The inputs provided to the function.
- Local Variables: Temporary variables used within the function.
- Return Address: The point in the code where execution should return once the function completes.
When a function calls another function, it's like entering a new level in the game. The game saves your progress at the current level before loading the next one. Likewise, the call stack pushes the current function's frame on top and creates a new frame for the called function. This ensures that each function has its own isolated environment to execute.
Understanding Stack Frames Through a Gaming Analogy
Let's imagine you're playing a role-playing game (RPG):
- Main Quest: You're exploring a town and decide to take on a hidden treasure quest. The game saves your current state at the town checkpoint.
- Hidden Treasure Quest: While completing the hidden treasure quest, you must enter a mysterious cave. The game saves your state for the hidden treasure quest before you enter the cave.
- Entering the Cave: You delve into the cave to find the treasure. The game saves this current state too.
In programming terms:
- Main Quest is your main function.
- Hidden Treasure Quest is the first function it calls (
hidden_treasure_quest()
). - Entering the Cave is the next function called by the previous one (
enter_cave()
).
Each "level" or function call is a stack frame on the call stack. When you complete a level or function, you return to the previous level, restoring the saved state.
Example of Function Calls
Consider this RPG-themed code example:
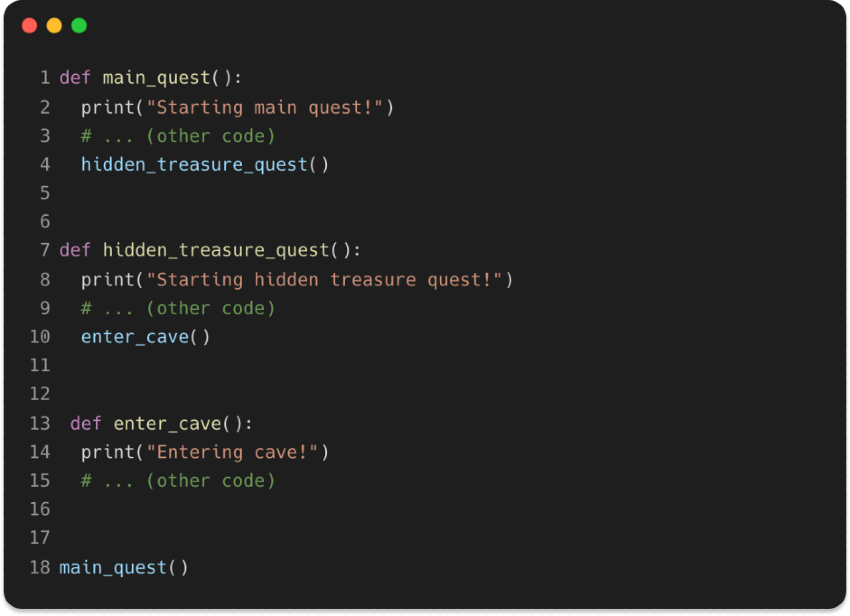
Here's how the call stack operates:
main_quest()
is called.- A stack frame is created for
main_quest()
. - The current state is saved.
- A stack frame is created for
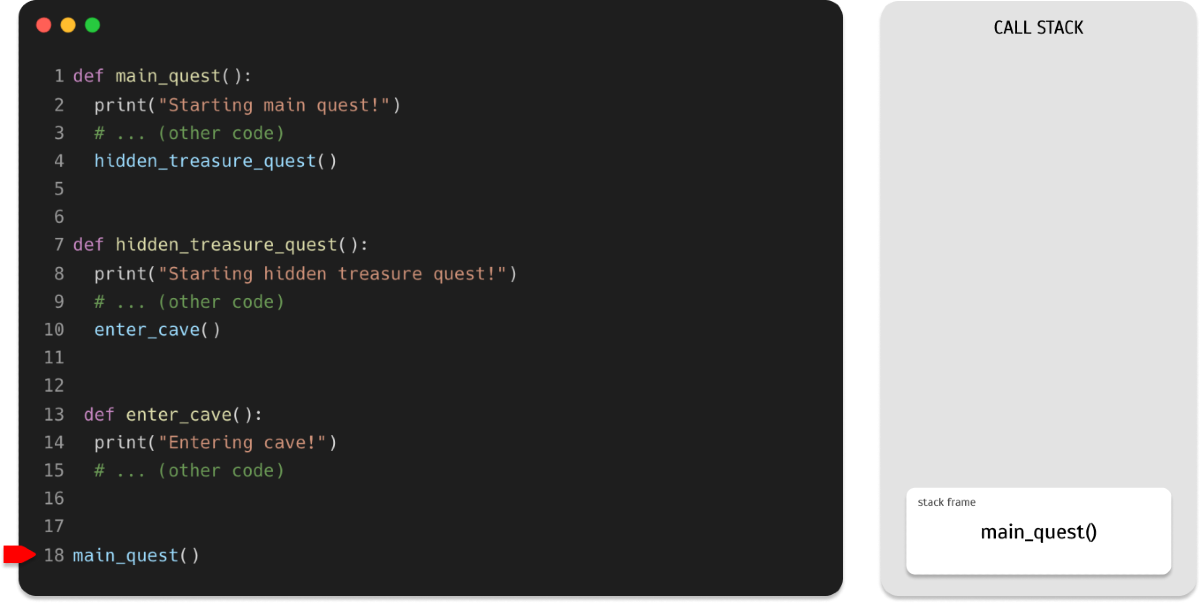
hidden_treasure_quest()
is invoked bymain_quest()
.- A new stack frame is pushed for
hidden_treasure_quest()
. - The execution context switches to
hidden_treasure_quest()
.
- A new stack frame is pushed for
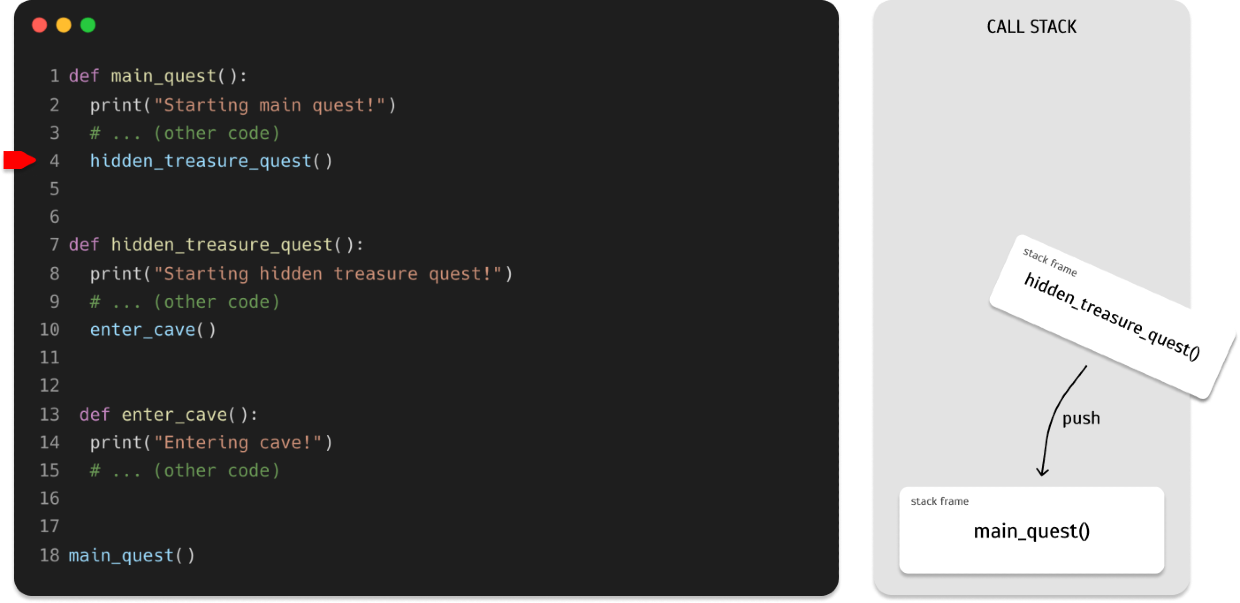
enter_cave()
is called byhidden_treasure_quest()
.- Another stack frame is pushed for
enter_cave()
. - The execution switches to
enter_cave()
.
- Another stack frame is pushed for
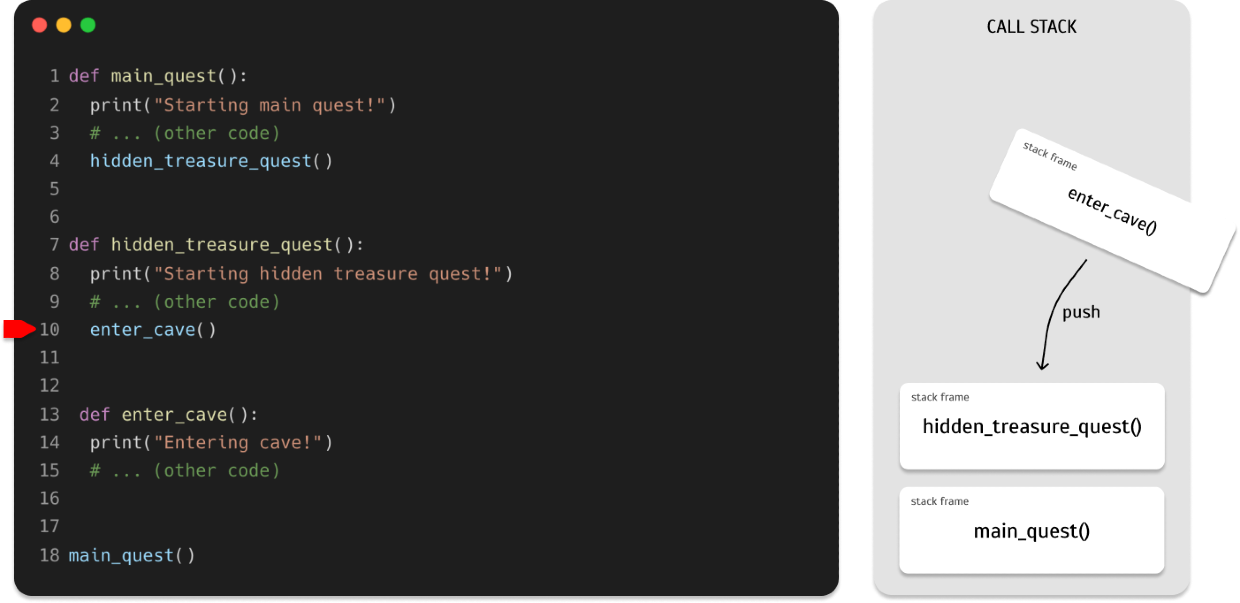
- Completion and Return:
- Once
enter_cave()
finishes, its frame is popped off the stack. - Control returns to
hidden_treasure_quest()
, and its context is restored. - When
hidden_treasure_quest()
completes, its frame is popped, returning control tomain_quest()
.
- Once
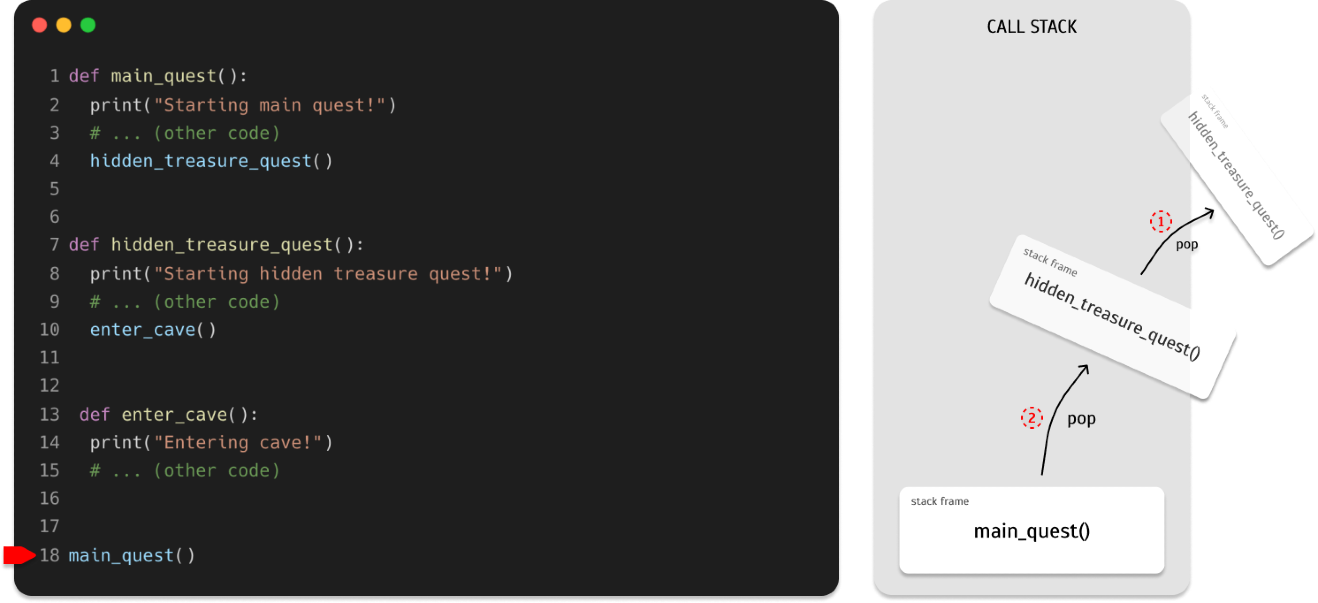
This process continues until all function calls are completed, and the call stack is empty.
Why Is the Call Stack Important?
The call stack is essential for:
- Managing Execution Order: Ensures functions are executed in the correct order and context.
- Handling Recursion: Supports functions that call themselves by maintaining separate frames for each recursive call.
- Debugging: Helps track where errors occur in the execution flow.
Understanding the call stack's operation and its role in managing function execution is crucial for grasping more advanced programming concepts, such as recursion and error handling.
Conclusion
In this article, we've embarked on a journey through the fascinating world of functions and their underlying mechanisms. We began by understanding the importance of functions in programming, emphasizing their role in promoting modularity, reusability, and maintainability. Functions allow developers to break down complex problems into smaller, more manageable tasks, making code easier to read, understand, and maintain.
Next, we delved into the intricacies of function calls, exploring how the call stack manages these operations under the hood. By drawing analogies to everyday experiences, such as stacking plates or navigating web pages, we uncovered how the stack structure operates on a Last In, First Out (LIFO) principle to track function execution order and context.
Using a video game analogy, we explored how stack frames save the current state of function execution, enabling smooth transitions between different functions as a program runs. This analogy helped illustrate how the call stack works to ensure functions are executed in the correct order and how it handles the completion and return of each function call.
Understanding the call stack is crucial for any programmer, as it lays the groundwork for more advanced topics, such as error handling and recursion. While we covered a lot of ground in this article, the call stack is just one piece of the puzzle.
What's Next?
In our next article, we'll take a deeper dive into recursion, a powerful programming technique that relies heavily on the call stack. Recursion involves functions that call themselves, allowing for elegant solutions to complex problems, but it also requires careful management to avoid pitfalls like infinite loops and stack overflows.
We'll explore how recursion works, examine practical examples, and discuss best practices for using recursion effectively in your code. By understanding recursion, you'll unlock new ways to think about problem-solving in programming, further enhancing your skills as a developer.
Stay tuned for the next article, where we'll unravel the mysteries of recursion and see how it builds upon the foundational knowledge we've gained about functions and the call stack.